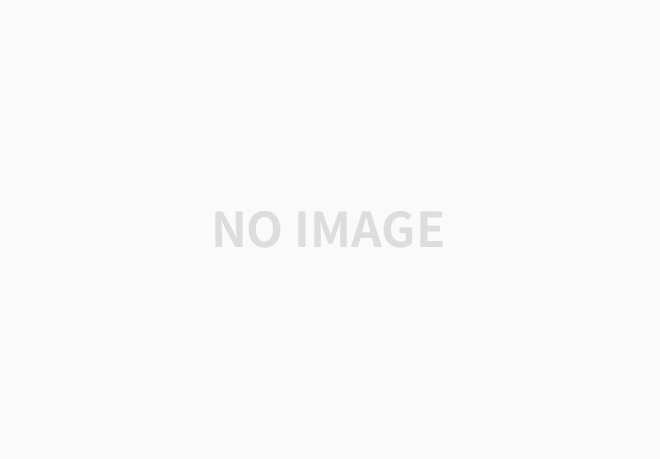
지금까지는 문자열을 char형의 배열로 선언했습니다.
하지만 C++부터는 string클래스를 이용해서 문자열을 저장해보겠습니다.
char배열과 가장 크게 달라진 점은 더 이상 배열의 크기를 신경 쓰지 않아도 된다는 점입니다.
먼저 string클래스를 사용하기위해서는 다음과 같은 새로운 헤더 파일을 추가해야 합니다.
#include <string>
string클래스에 문자열을 초기화하는 방법은 다음과 같습니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str = "Hello World";
cout << str;
return 0;
}
string은 클래스임으로 객체를 생성하는 방법과 같습니다.
그래서 다음과 같은 방법으로도 초기화가 가능합니다.
string str("Hello World");
char배열은 strcpy()를 사용해서 문자열을 새롭게 대입했습니다.
하지만 string은 단순히 =(대입연사자)만으로도 새롭게 문자열을 대입할 수 있습니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str("Hello World");
cout << str << '\n';
str = "World";
cout << str << '\n';
return 0;
}
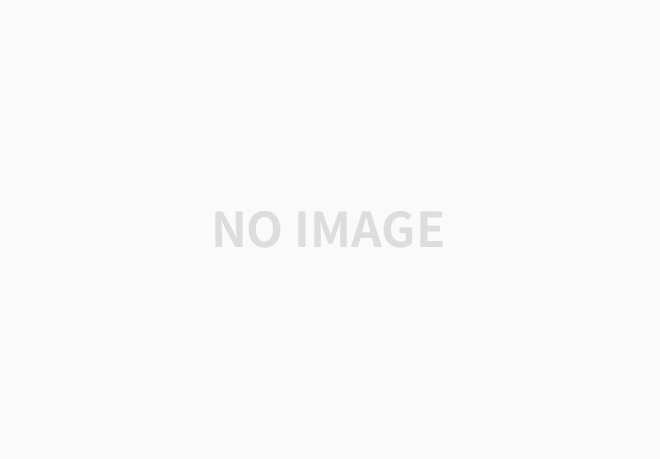
문자열과 문자열을 연결할때도 단순하게 +연산자만으로 연결이 가능합니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1("Hello World");
string str2("안녕하세요");
str1 += str2;
cout << str1 << '\n';
return 0;
}
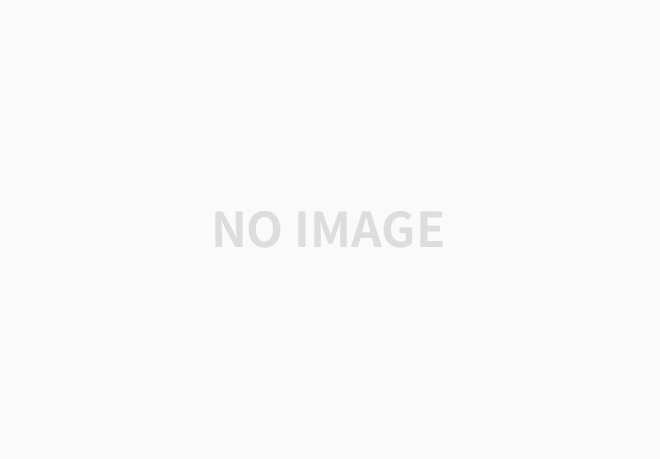
string은 char의 배열처럼 인덱스를 사용할 수 있습니다.
인덱스를 이용해 원하는 위치의 특정 문자를 가져오거나 수정할 수 있습니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
//string str = "Hello World";
string str("Hello World");
str[1] = 'i';
cout << str[1] << '\n';
return 0;
}
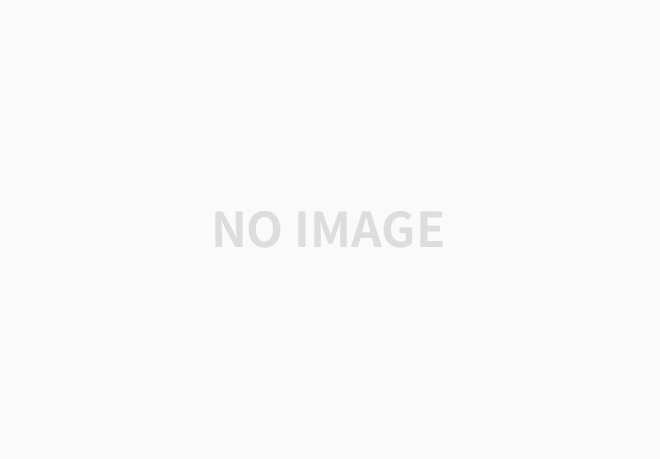
string은 클래스이다 보니 많은 메서드를 지원합니다.
많이 사용하는 몇가지를 알아보겠습니다.
length()
문자열의 총 길이를 반환합니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = "Hello World";
cout <<"문자열의 길이는 : "<< str1.length()<<'\n';
return 0;
}
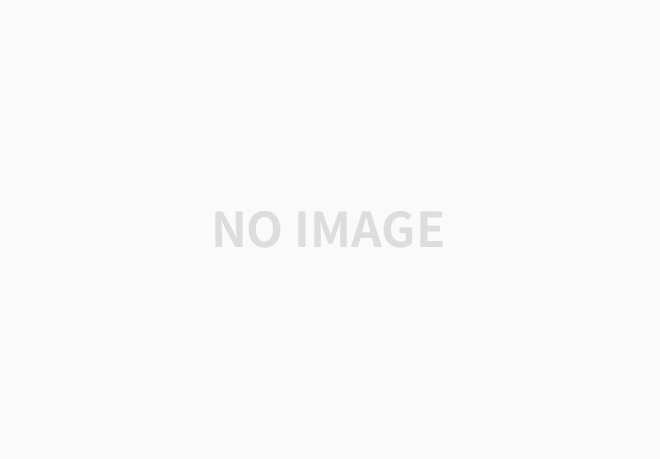
append()
해당 string객체에 문자열을 연결해줍니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = "Hello World";
string str2 = "안녕하세요";
str1.append(str2);
str1.append("C++");
cout << str1 <<'\n';
return 0;
}
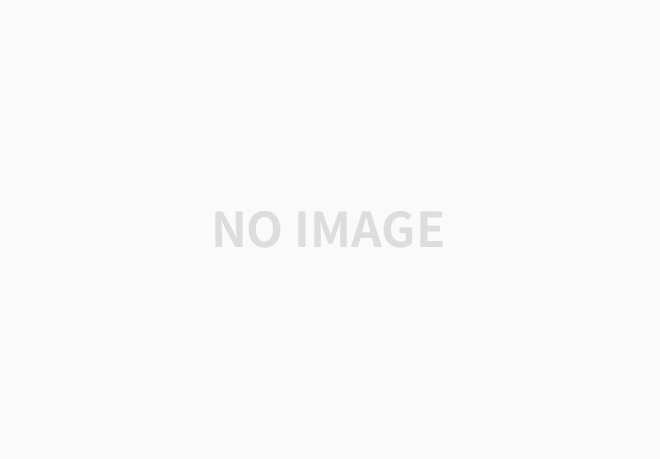
find()
특정 문자 또는 문자열을 찾아서 시작 인덱스를 반환합니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = "Hello World";
cout << str1.find('o') <<'\n';
cout << str1.find("Wo") << '\n';
return 0;
}
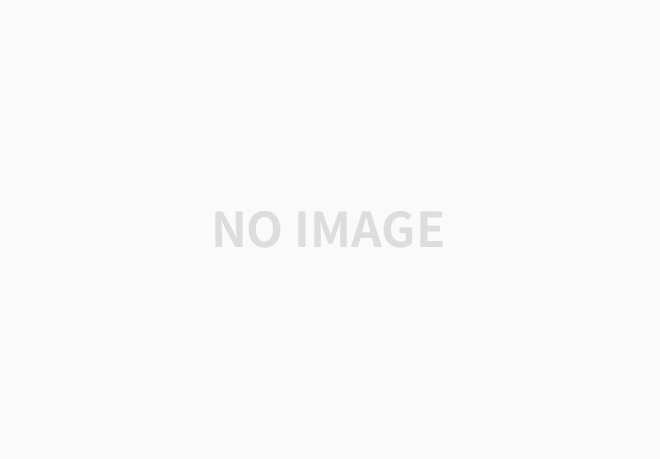
insert()
입력된 문자열을 지정된 인덱스부터 삽입합니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = "Hello World";
str1.insert(7, "안녕하세요");
cout << str1 <<'\n';
return 0;
}
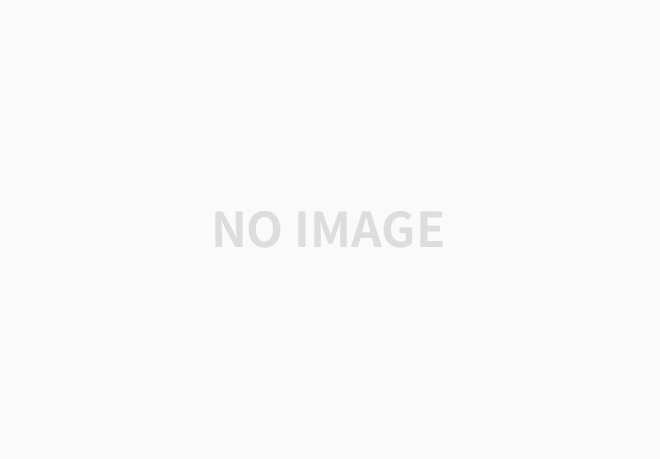
clear()
저장된 문자열을 모두 삭제해줍니다.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string str1 = "Hello World";
str1.clear();
cout << str1 <<'\n';
return 0;
}
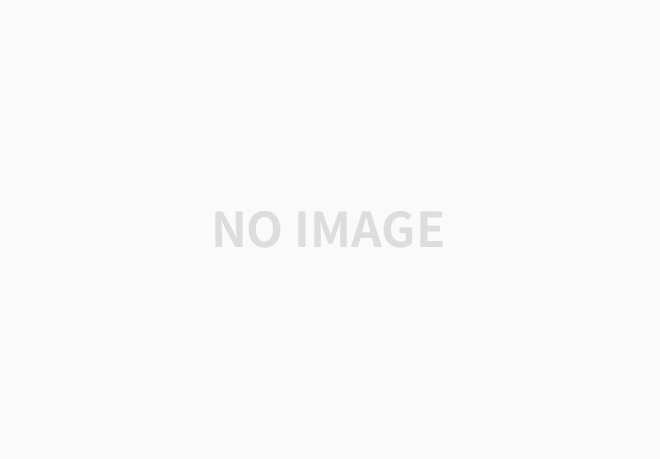
이밖에도 다양한 메서드가 있습니다.
'공부 자료실 > C++' 카테고리의 다른 글
C++ - 클래스(class) 상속 (0) | 2020.08.21 |
---|---|
C++ - 클래스(class) 생성자와 소멸자 (0) | 2020.08.19 |
C++ - 클래스(Class)의 구조와 접근 지정자 (0) | 2020.08.17 |
C++ - 네임스페이스(namespace) (0) | 2020.08.13 |
C++ - 입출력 (0) | 2020.08.12 |
댓글